This repository has been archived by the owner on Mar 1, 2024. It is now read-only.
-
Notifications
You must be signed in to change notification settings - Fork 736
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Jarod Stewart
authored
Feb 7, 2024
1 parent
d08422a
commit b066238
Showing
9 changed files
with
343 additions
and
9 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,43 @@ | ||
# Ionic Shopping Tool | ||
|
||
[Ionic](https://ioniccommerce.com) is a plug and play ecommerce marketplace for AI Assistants. | ||
By including the Ionic Tool in your agent, you are effortlessly providing your users with the ability | ||
to shop and transact directly within your agent, and you’ll get a cut of the transaction. | ||
|
||
## Attribution | ||
Llearn more about how [Ionic attributes sales](https://docs.ioniccommerce.com/guides/attribution) | ||
to your agent. Provide your Ionic API Key when instantiating the tool: | ||
```python | ||
from llama_hub.tools.ionic_shopping.base import IonicShoppingToolSpec | ||
|
||
ionic_tool = IonicShoppingToolSpec(api_key="<my Ionic API Key>").to_tool_list() | ||
``` | ||
|
||
|
||
## Usage | ||
Try it out using the [Jupyter notebook](https://github.com/run-llama/llama-hub/blob/main/llama_hub/tools/notebooks/ionic_shopping.ipynb). | ||
|
||
```python | ||
import openai | ||
from llama_index.agent import OpenAIAgent | ||
from llama_hub.tools.ionic_shopping.base import IonicShoppingToolSpec | ||
|
||
openai.api_key = "sk-api-key" | ||
|
||
ionic_tool = IonicShoppingToolSpec(api_key="<my Ionic API Key>").to_tool_list() | ||
|
||
agent = OpenAIAgent.from_tools(ionic_tool) | ||
print( | ||
agent.chat( | ||
"I'm looking for a 5k monitor can you find me 3 options between $600 and $1000" | ||
) | ||
) | ||
``` | ||
|
||
`query`: used to search for products and to get product recommendations | ||
|
||
|
||
Your users can use natural language to specify how many results they would like to see | ||
and what their budget is. | ||
|
||
For more information on setting up your Agent with Ionic, see the [Ionic documentation](https://docs.ioniccommerce.com). |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,6 @@ | ||
"""Ionic Shopping Tool""" | ||
from llama_hub.tools.ionic_shopping.base import ( | ||
IonicShoppingToolSpec, | ||
) | ||
|
||
__all__ = ["IonicShoppingToolSpec"] |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,63 @@ | ||
from __future__ import annotations | ||
from typing import Optional | ||
from llama_index.tools.tool_spec.base import BaseToolSpec | ||
|
||
from ionic.models.components import Product, Query as SDKQuery, QueryAPIRequest | ||
from ionic.models.operations import QueryResponse, QuerySecurity | ||
|
||
|
||
class IonicShoppingToolSpec(BaseToolSpec): | ||
"""Ionic Shopping tool spec | ||
This tool can be used to build e-commerce experiences with LLMs. | ||
""" | ||
|
||
spec_functions = ["query"] | ||
|
||
def __init__(self, api_key: Optional[str] = None) -> None: | ||
"""Ionic API Key | ||
Learn more about attribution with Ionic API Keys | ||
https://docs.ioniccommerce.com/guides/attribution | ||
""" | ||
from ionic import Ionic as IonicSDK | ||
|
||
if api_key: | ||
self.client = IonicSDK(api_key_header=api_key) | ||
else: | ||
self.client = IonicSDK() | ||
|
||
def query( | ||
self, | ||
query: str, | ||
num_results: Optional[int] = 5, | ||
min_price: Optional[int] = None, | ||
max_price: Optional[int] = None, | ||
) -> list[Product]: | ||
""" | ||
Use this function to search for products and to get product recommendations | ||
Args: | ||
query (str): A precise query of a product name or product category | ||
num_results (Optional[int]): Defaults to 5. The number of product results to return. | ||
min_price (Option[int]): The minimum price in cents the requester is willing to pay | ||
max_price (Option[int]): The maximum price in cents the requester is willing to pay | ||
""" | ||
request = QueryAPIRequest( | ||
query=SDKQuery( | ||
query=query, | ||
num_results=num_results, | ||
min_price=min_price, | ||
max_price=max_price, | ||
) | ||
) | ||
response: QueryResponse = self.client.query( | ||
request=request, | ||
security=QuerySecurity(), | ||
) | ||
|
||
return [ | ||
product | ||
for result in response.query_api_response.results | ||
for product in result.products | ||
] |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1 @@ | ||
ionic-api-sdk |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,205 @@ | ||
{ | ||
"cells": [ | ||
{ | ||
"cell_type": "markdown", | ||
"source": [ | ||
"# Ionic Shopping Tool\n", | ||
"\n", | ||
"[Ionic](https://www.ioniccommerce.com/) is a plug and play ecommerce marketplace for AI Assistants. By using Ionic, you are effortlessly providing your users with the ability to shop and transact directly within your agent, and you'll get a cut of the transaction.\n", | ||
"\n", | ||
"This is a basic jupyter notebook demonstrating how to integrate the Ionic Shopping Tool. For more information on setting up your Agent with Ionic, see the Ionic [documentation](https://docs.ioniccommerce.com/introduction).\n", | ||
"\n", | ||
"This Jupyter Notebook demonstrates how to use the Ionic tool with an Agent.\n", | ||
"\n", | ||
"---\n", | ||
"\n", | ||
"## Setup the Tool\n", | ||
"### First, let's install our dependencies" | ||
], | ||
"metadata": { | ||
"collapsed": false | ||
}, | ||
"id": "8024471faac3a296" | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"outputs": [], | ||
"source": [ | ||
"!pip install llama-index llama-hub ionic-api-sdk" | ||
], | ||
"metadata": { | ||
"collapsed": false | ||
}, | ||
"id": "856d9803ec1cdd9c", | ||
"execution_count": null | ||
}, | ||
{ | ||
"cell_type": "markdown", | ||
"source": [ | ||
"### Configure OpenAI" | ||
], | ||
"metadata": { | ||
"collapsed": false | ||
}, | ||
"id": "eedd002505e12801" | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"execution_count": 2, | ||
"id": "initial_id", | ||
"metadata": { | ||
"collapsed": true, | ||
"ExecuteTime": { | ||
"end_time": "2024-01-29T22:46:08.765992Z", | ||
"start_time": "2024-01-29T22:46:07.725961Z" | ||
} | ||
}, | ||
"outputs": [], | ||
"source": [ | ||
"import openai\n", | ||
"from llama_index.agent import OpenAIAgent\n", | ||
"\n", | ||
"openai.api_key = \"sk-api-key\"" | ||
] | ||
}, | ||
{ | ||
"cell_type": "markdown", | ||
"source": [ | ||
"### Import and configure the Ionic Shopping Tool " | ||
], | ||
"metadata": { | ||
"collapsed": false | ||
}, | ||
"id": "8506dd2f8e36cad4" | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"outputs": [ | ||
{ | ||
"name": "stdout", | ||
"output_type": "stream", | ||
"text": [ | ||
"query\n" | ||
] | ||
} | ||
], | ||
"source": [ | ||
"from llama_hub.tools.ionic_shopping.base import IonicShoppingToolSpec\n", | ||
"\n", | ||
"# optionally add you Ionic API Key\n", | ||
"# IonicShoppingToolSpec(api_key=\"<my Ionic API Key>\")\n", | ||
"ionic_tool = IonicShoppingToolSpec().to_tool_list()\n", | ||
"\n", | ||
"for tool in ionic_tool:\n", | ||
" print(tool.metadata.name)" | ||
], | ||
"metadata": { | ||
"collapsed": false, | ||
"ExecuteTime": { | ||
"end_time": "2024-01-29T22:46:08.779746Z", | ||
"start_time": "2024-01-29T22:46:08.766351Z" | ||
} | ||
}, | ||
"id": "f95ef80d8aa9bd5f", | ||
"execution_count": 3 | ||
}, | ||
{ | ||
"cell_type": "markdown", | ||
"source": [ | ||
"### Use Ionic" | ||
], | ||
"metadata": { | ||
"collapsed": false | ||
}, | ||
"id": "80b4d128986d4a9" | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"outputs": [], | ||
"source": [ | ||
"agent = OpenAIAgent.from_tools(\n", | ||
" ionic_tool,\n", | ||
")" | ||
], | ||
"metadata": { | ||
"collapsed": false, | ||
"ExecuteTime": { | ||
"end_time": "2024-01-29T22:46:08.935312Z", | ||
"start_time": "2024-01-29T22:46:08.780878Z" | ||
} | ||
}, | ||
"id": "18bd589c4fd6ef44", | ||
"execution_count": 4 | ||
}, | ||
{ | ||
"cell_type": "code", | ||
"outputs": [ | ||
{ | ||
"name": "stdout", | ||
"output_type": "stream", | ||
"text": [ | ||
"Query Query(query='5k monitor', max_price=100000, min_price=60000, num_results=3)\n", | ||
"Here are 3 options for a 5k monitor between $600 and $1000:\n", | ||
"\n", | ||
"1. SAMSUNG 27\" ViewFinity S9 Series 5K Computer Monitor - $999.99\n", | ||
" - [Details](https://www.amazon.com/dp/B0CB71BY87?tag=ioniccommer00-20&linkCode=osi&th=1&psc=1)\n", | ||
" - \n", | ||
" - Brand: SAMSUNG\n", | ||
" - Available on Amazon\n", | ||
"\n", | ||
"2. SAMSUNG 27\" Class QHD Curved Monitor - $699.99\n", | ||
" - [Details](https://brwi.short.gy/qxJVZ6)\n", | ||
" - 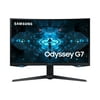\n", | ||
" - Brand: Samsung\n", | ||
" - UPC: 887276413129\n", | ||
" - Available at Walmart\n", | ||
"\n", | ||
"3. SAMSUNG 27\" ViewFinity S9 Series 5K Computer Monitor (Open Box) - $749.99\n", | ||
" - [Details](https://brwi.short.gy/YAYBuD)\n", | ||
" - \n", | ||
" - Available on eBay\n", | ||
"\n", | ||
"Please let me know if you need more information about any of these options.\n" | ||
] | ||
} | ||
], | ||
"source": [ | ||
"print(\n", | ||
" agent.chat(\n", | ||
" \"I'm looking for a 5k monitor can you find me 3 options between $600 and $1000\"\n", | ||
" )\n", | ||
")" | ||
], | ||
"metadata": { | ||
"collapsed": false, | ||
"ExecuteTime": { | ||
"end_time": "2024-01-29T22:46:55.891101Z", | ||
"start_time": "2024-01-29T22:46:37.542833Z" | ||
} | ||
}, | ||
"id": "aa6ee6bd0b1c8f22", | ||
"execution_count": 6 | ||
} | ||
], | ||
"metadata": { | ||
"kernelspec": { | ||
"display_name": "Python 3", | ||
"language": "python", | ||
"name": "python3" | ||
}, | ||
"language_info": { | ||
"codemirror_mode": { | ||
"name": "ipython", | ||
"version": 2 | ||
}, | ||
"file_extension": ".py", | ||
"mimetype": "text/x-python", | ||
"name": "python", | ||
"nbconvert_exporter": "python", | ||
"pygments_lexer": "ipython2", | ||
"version": "2.7.6" | ||
} | ||
}, | ||
"nbformat": 4, | ||
"nbformat_minor": 5 | ||
} |
Oops, something went wrong.